1. VPython(Glowscript)을 이용한 3차원 물체 표현
좌표계
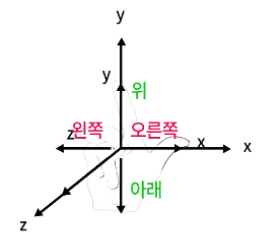
GlowScript 2.9 VPython
#Creating Ball
myBall = sphere(color=color.red, radius=2)
#Creating Box
myBox = box(pos = vec(5,0,0), color = color.blue, size = vec(0.5,4,1))
myBall.color = color.green
myBox.pos.x = 10
2. VPython(Glowscript)을 이용한 벡터 연산
- 벡터는 크기(magnitude)와 방향(direction)을 갖는다.
선의 길이는 크기를 나타내고 화살표는 방향을 나타낸다.
- 다양한 벡터들 : 속도(velocity), 가속도(acceleration), 힘(force)
- 두 벡터는 더할 수 있다.
- 두 벡터를 뺄 수도 있다.
- 벡터의 표기법 : 굵은 a
- 벡터 a는 두개의 벡터 ax 와 ay 로 나눌 수 있다.
a = (ax, ay)
- 벡터 더하기 예제
a = (8, 13) , b = (26, 7)
c = a + b
c = (8, 13) + (26, 7) = (8+26, 13+7) = (34, 20)
- 벡터 빼기 예제
v = (12, 2) , k = (4, 5)
a = v + −k
a = (12, 2) + −(4, 5) = (12, 2) + (−4, −5) = (12−4, 2−5) = (8, −3)
- 벡터의 크기 표기법
|a|
또는, 절대값과 혼돈되지 않도록 ||a||
- 벡터의 크기 계산
피타고라스의 정리
|a| = √( x2 + y2 )
벡터 b의 크기는 ?
b = (6, 8)
|b| = √( 62 + 82) = √( 36+64) = √100 = 10
스칼라 : 크기만 표기
스칼라값 계산 예제
GlowScript 3.0 VPython
# Vectors
r= vector(6,8,0)
# 벡터의 크기 : 스칼라값
mag_val = sqrt(6**2 + 8**2)
print("Magnitude:", mag_val)
r_mag = mag(r)
print("mag(r):", r_mag)
- 단위벡터
Unit Vector
크기가 1인 벡터
표기법 : a “hat“
스케일링 : 단위벡터의 2.5배
벡터의 크기와 방향
GlowScript 2.9 VPython
#Vectors
r= vector(3,4,5)
r_arrow = arrow(pos = vector(0,0,0), axis=r, shafrwidth=0.2)
#Axes (사물의) (중심) 축, 축선
x_axis = arrow(axis=vector(10,0,0), color=color.red, shaftwidth=0.1)
y_axis = arrow(axis=vector(0,10,0), color=color.green, shaftwidth=0.1)
z_axis = arrow(axis=vector(0,0,10), color=color.blue, shaftwidth=0.1)
r_mag = mag(r) // 벡터의 크기 : 스칼라값
r_hat = norm(r) // 벡터의 방향 : 단위벡터
r_hat_arrow = arrow(axis=r_hat, color=color.cyan, shaftwidth=0.2)
print("r:", r)
print("r_mag:", r_mag)
print("r_hat:", r_hat)
mag(r)
- 벡터 r의 크기(길이)를 계산해서 반환하는 함수
norm(r)
- 벡터 r의 단위 벡터를 계산해서 반환하는 함수
벡터 연산
GlowScript 3.0 VPython
a = vector(3,4,0)
b = vector(5,1,0)
# 벡터더하기
c = a + b
# 벡터빼기
d = a - b
# 벡터의내적
e = dot(a,b)
deg = acos(e/mag(a)/mag(b)) ## rad
deg = degrees(deg) ## deg
a_arrow = arrow(pos=vector(0,0,0), axis=a, shaftwidth=0.2,color=color.blue)
b_arrow = arrow(pos=vector(0,0,0), axis=b, shaftwidth=0.2,color=color.green)
#c_arrow = arrow(pos=vector(0,0,0), axis=c, shaftwidth=0.2,color=color.red)
d_arrow = arrow(pos=b, axis=d, shaftwidth=0.2,color=color.red)
print("a+b=", c)
print("a-b=", d)
print("dot(a,b) =", e)
print("degree(a,b) =", deg)
- 두 벡터 곱하기
벡터의 내적 : Dot Product : 결과값 스칼라
벡터의 외적 : Cross Product : 결과값 벡터
벡터내적 : Dot Product
GlowScript 2.9 VPython
#Vectors
v = vector(3,4,0)
r = vector(1,0,0)
v_par = dot(v,r)*r
v_per = v - v_par
v_arr = arrow(axis=v, shaftwidth=0.2)
r_arr = arrow(axis=r, shaftwidth=-0.3, color=color.blue)
v_par_arr = arrow(axis=v_par, shaftwidth=0.2, color= color.green)
v_per_arr = arrow(axis=v_per, shaftwidth=0.2, color= color.yellow)
print("v=", v)
print("r=", r)
print("dot(v,r)=", dot(v,r))
print("v_par=", v_par)
print("v_per=", v_per)
벡터의 외적 : Cross Product
GlowScript 2.9 VPython
#Vectors
a = vector(1,2,3)
b = vector(-1,2,0)
c = cross(a,b)
a_arr = arrow(axis=a, shaftwidth=0.1, color=color.red)
b_arr = arrow(axis=b, shaftwidth=0.1, color=color.blue)
c_arr = arrow(axis=c, shaftwidth=0.1)
d = cross(b,a)
d_arr = arrow(axis=d, shaftwidth=0.1, color=color.yellow)